Cart 0
Arduino Distance Measurement with LCD
In this project, I’ve built a simple Arduino-based system that measures the distance to an object using an ultrasonic sensor and displays the results on a 16×2 I2C LCD. This project demonstrates the versatility of Arduino in combining multiple components like sensors and displays for real-world applications. The system calculates distance in real-time and categorizes objects as “Close” or “Far” based on a user-defined threshold, making it ideal for educational purposes, robotics, or DIY home automation projects. The combination of straightforward code and accessible components ensures this project is beginner-friendly and fun to build!
Circuit Diagram
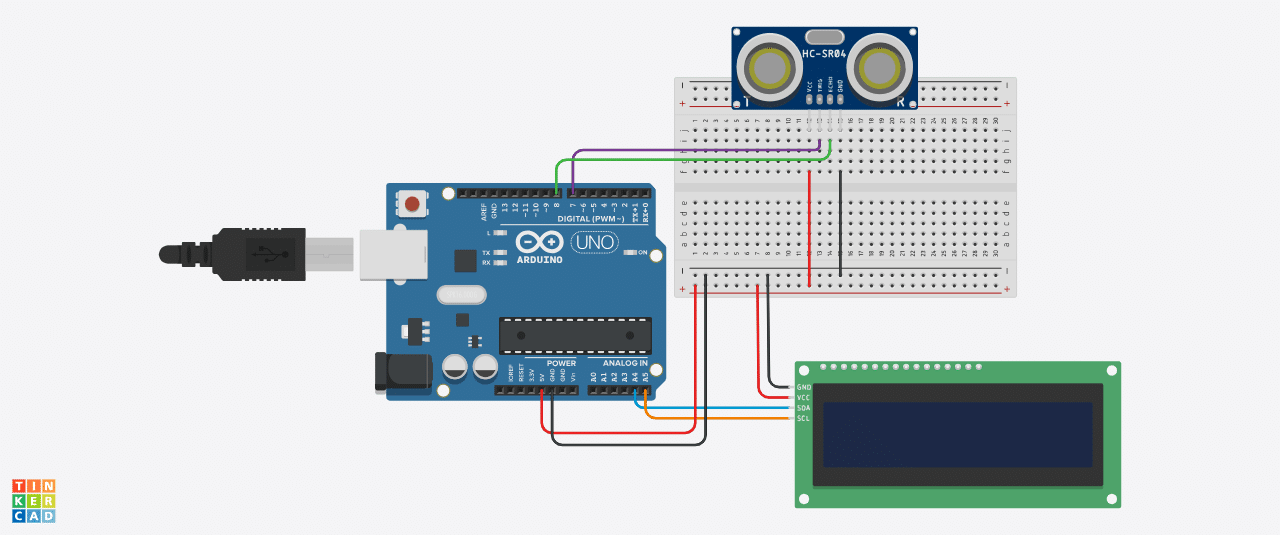
Code
#include <Wire.h> // Include Wire library for I2C
#include <LiquidCrystal_I2C.h> // Include library for LCD I2C
#define TRIG_PIN 7 // Pin for the ultrasonic sensor's trig pin
#define ECHO_PIN 8 // Pin for the ultrasonic sensor's echo pin
// Set up the LCD display with the I2C address (typically 0x27 or 0x3F)
LiquidCrystal_I2C lcd(0x27, 16, 2); // 16x2 LCD
void setup() {
pinMode(TRIG_PIN, OUTPUT);
pinMode(ECHO_PIN, INPUT);
lcd.init(); // Initialize the LCD
lcd.backlight(); // Turn on the backlight
lcd.print("Initializing");
delay(2000);
lcd.clear();
}
void loop() {
long duration;
float distance;
// Send a 10us pulse to trigger the ultrasonic sensor
digitalWrite(TRIG_PIN, LOW);
delayMicroseconds(2);
digitalWrite(TRIG_PIN, HIGH);
delayMicroseconds(10);
digitalWrite(TRIG_PIN, LOW);
// Read the echo pin and calculate the distance
duration = pulseIn(ECHO_PIN, HIGH);
distance = (duration * 0.034) / 2; // Convert to cm
// Display the distance on the LCD
lcd.setCursor(0, 0);
lcd.print("Distance: ");
lcd.print(distance);
lcd.print(" cm");
// Determine if the object is close or far
lcd.setCursor(0, 1);
if (distance < 20) { // Adjust the threshold as needed
lcd.print("Close "); // Ensure the display clears extra characters
} else {
lcd.print("Far "); // Ensure the display clears extra characters
}
delay(500); // Delay for readability and to reduce flicker
}